Java Predicate 결합. CF와 함수형 인터페이스
by 볼빵빵오춘기Predicate의 결합
※ predicate = 조건식
- and(), or(), negate()로 두 Predicate를 하나로 결합한다.(default메서드 - static, 추상메서드도 가질 수 있음)
- 등가비교를 위한 Predicate의 작성에는 isEqual()를 사용한다. (static 메서드)
예제 코드
더보기

import java.util.function.*;
class Ex14_3 {
public static void main(String[] args) {
Function<String, Integer> f = (s) -> Integer.parseInt(s, 16);
Function<Integer, String> g = (i) -> Integer.toBinaryString(i);
Function<String, String> h = f.andThen(g);
Function<Integer, Integer> h2 = f.compose(g);
System.out.println(h.apply("FF")); // "FF" → 255 → "11111111"
System.out.println(h2.apply(2)); // 2 → "10" → 16
Function<String, String> f2 = x -> x; // 항등 함수(identity function)
System.out.println(f2.apply("AAA")); // AAA가 그대로 출력됨
Predicate<Integer> p = i -> i < 100;
Predicate<Integer> q = i -> i < 200;
Predicate<Integer> r = i -> i%2 == 0;
Predicate<Integer> notP = p.negate(); // i >= 100
Predicate<Integer> all = notP.and(q.or(r));
System.out.println(all.test(150)); // true
String str1 = "abc";
String str2 = "abc";
// str1과 str2가 같은지 비교한 결과를 반환
Predicate<String> p2 = Predicate.isEqual(str1);
boolean result = p2.test(str2);
System.out.println(result);
}
}

컬렉션 프레임웍과 함수형 인터페이스
함수형 인터페이스를 사용하는 컬렉션 프레임웍의 메서드이다.(와일드 카드 생략)
예제 코드
더보기
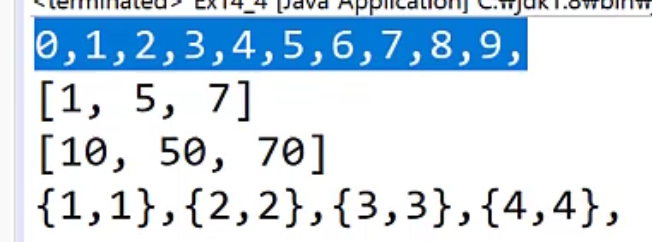
import java.util.*;
class Ex14_4 {
public static void main(String[] args) {
ArrayList<Integer> list = new ArrayList<>();
for(int i=0;i<10;i++)
list.add(i);
// list의 모든 요소를 출력
list.forEach(i->System.out.print(i+","));
System.out.println();
// list에서 2 또는 3의 배수를 제거한다.
list.removeIf(x-> x%2==0 || x%3==0);
System.out.println(list);
list.replaceAll(i->i*10); // list의 각 요소에 10을 곱한다.
System.out.println(list);
Map<String, String> map = new HashMap<>();
map.put("1", "1");
map.put("2", "2");
map.put("3", "3");
map.put("4", "4");
// map의 모든 요소를 {k,v}의 형식으로 출력한다.
map.forEach((k,v)-> System.out.print("{"+k+","+v+"},"));
System.out.println();
}
}
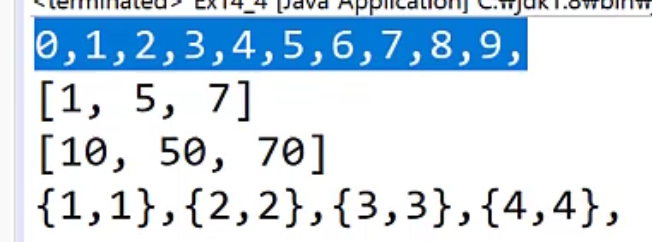
'👩🏻💻 About 프로그래밍 > Java' 카테고리의 다른 글
Java 스트림(Stream), 스트림의 특징 (0) | 2023.12.11 |
---|---|
Java 메서드 참조, 생성자의 메서드 참조 (0) | 2023.12.11 |
Java java.util.function 패키지 (0) | 2023.12.11 |
Java 함수형 인터페이스 (0) | 2023.12.11 |
Java 람다식(Lambda Expression) (0) | 2023.12.11 |
블로그의 정보
Hello 춘기's world
볼빵빵오춘기