Java 쓰레드의 실행제어 메서드
by 볼빵빵오춘기더보기
Thread실행 제어 - static이 붙은 메서드
- sleep()
- yiled()
⇒ 자기자신
sleep()
- 현재 쓰레드를 지정된 시간동안 멈추게 한다.
- 예외처리를 해야 한다.(InterruptedException이 발생하면 깨어난다.)
- 특정 쓰레드를 지정해서 멈추게 하는 것은 불가능하다.
예제 코드
더보기
public class Try {
public static void main(String[] args) {
ThreadEx8_1 th1 = new ThreadEx8_1();
ThreadEx8_2 th2 = new ThreadEx8_2();
th1.start(); th2.start();
// => main스레드가 잠자게 되는것
// 주석1 start
// try {
// th1.sleep(2000); // th1을 2초동안 잠자게
// Thread.sleep(2000); // th1을 2초동안 잠자게
// } catch(InterruptedException e) {}
// 주석1 end
//------------------------------
// 주석2 start
// try {
// th1.sleep(2000); // th1을 2초동안 잠자게
// Thread.sleep(2000); // th1을 2초동안 잠자게
// } catch(InterruptedException e) {}
// 주석2 end
System.out.print("<<main 종료>>");
}
}
class ThreadEx8_1 extends Thread {
public void run() {
for(int i=0; i < 300; i++) System.out.print("-");
System.out.print("<<th1 종료>>");
} // run()
}
class ThreadEx8_2 extends Thread {
public void run() {
for(int i=0; i < 300; i++) System.out.print("|");
System.out.print("<<th2 종료>>");
} // run()
}
결과값 - 주석1처리한 후, 주석2처리한 후
|||||||||||||||||||||||||||||||||||||||||||||<<main 종료>>--|||||||||||||||||||||||||||||||--------------------------------------------------------------------------------|||||||||||||||||||||---------||||||||||||||--------------|||||||||||||||||||||||||||||||||||||||||||||||||||||||||||-|-------------------------------------------------------------------------------------------------------------------------------------------------||||||||||||||||||||||||||||------||||||---------------||||||-----------|||||||||||||---||||||||||||------------||||||||||||||||||||||||||||||||--<<th1 종료>>||||||||||||||||||||||||||||||||<<th2 종료>>
결과값 - 주석1처리푼 후 , 주석2처리한 후
-------------------||||||||||||||||||----------------------------------------------------|||||-------------||||||||||||||||||||||----|-|-----------------------------------------------||||||-||------------------------|||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||--------------------------------------------------------------------|||-|||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||------------------------------------------------||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||----<<th2 종료>>-----------------<<th1 종료>><<main 종료>>
결과값 - 주석1처리한 후, 주석2처리푼 후
-----------------------------------------|||||||||||||||||||||||||||||||||||||||||||||||||||||-----------------|-------------------------|--||-|---------------|-------|-|||-|||||||-|--||||||||||-------|-|-|----------------------------------------------------------------------------------------------------------------------------------------------|||||||||||||||||||||||||||||||||||||||||||||||||||||||||-----------------------------------<<th1 종료>>|||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||<<th2 종료>>
interrupt()
대기상태(waiting)인 쓰레드를 실행대기 상태(runnable)로 만든다.
예제 코드
더보기

import javax.swing.JOptionPane;
public class Try {
public static void main(String[] args) {
ThreadEx9_1 th1 = new ThreadEx9_1();
th1.start();
String input = JOptionPane.showInputDialog("아무 값이나 입력하세요.");
System.out.println("입력하신 값은 " + input + "입니다.");
th1.interrupt(); // interrupt()를 호출하면, interrupted상태가 true가 된다.
System.out.println("isInterrupted():"+ th1.isInterrupted()); // true
}
}
class ThreadEx9_1 extends Thread {
public void run() {
int i = 10;
while(i!=0 && !isInterrupted()) {
System.out.println(i--);
for(long x=0;x<2500000000L;x++); // 시간 지연
}
System.out.println("카운트가 종료되었습니다.");
}
}

suspend(), resume(), stop()
- 쓰레드의 실행을 일시정지, 재개, 완전정지 시킨다.
쓰면 편하나 suspend(), resume(), stop()이 deprecated 되었다.
더보기

🤔 why? dead-lock 교착상태때문에

⇒ 우리가 직접 구현(작성)할 수 있다.
예제 코드
더보기

import javax.swing.JOptionPane;
public class Try {
public static void main(String[] args) {
RunImplEx10 r = new RunImplEx10();
Thread th1 = new Thread(r, "*");
Thread th2 = new Thread(r, "**");
Thread th3 = new Thread(r, "***");
th1.start();
th2.start();
th3.start();
try {
Thread.sleep(2000);
th1.suspend(); // 쓰레드 th1을 잠시 중단시킨다.
Thread.sleep(2000);
th2.suspend();
Thread.sleep(3000);
th1.resume(); // 쓰레드 th1이 다시 동작하도록 한다.
Thread.sleep(3000);
th1.stop(); // 쓰레드 th1을 강제종료시킨다.
th2.stop();
Thread.sleep(2000);
th3.stop();
} catch (InterruptedException e) {}
}
}
class RunImplEx10 implements Runnable {
public void run() {
while(true) {
System.out.println(Thread.currentThread().getName());
try {
Thread.sleep(1000);
} catch(InterruptedException e) {}
}
} // run()
}

⇒ 1줄 출력되고 시간차를 두고 차근차근나온다.
⇒ 별표 순서는 상관없이 출력이 된다.(쓰레드의 특정. os스케쥴러가 관리)
join()
- 지정된 시간동안 특정 쓰레드가 작업하는 것을 기다린다.
- 예외처리를 해야 한다.(InterruptedException이 발생하면 작업 재개)
예제 코드
더보기

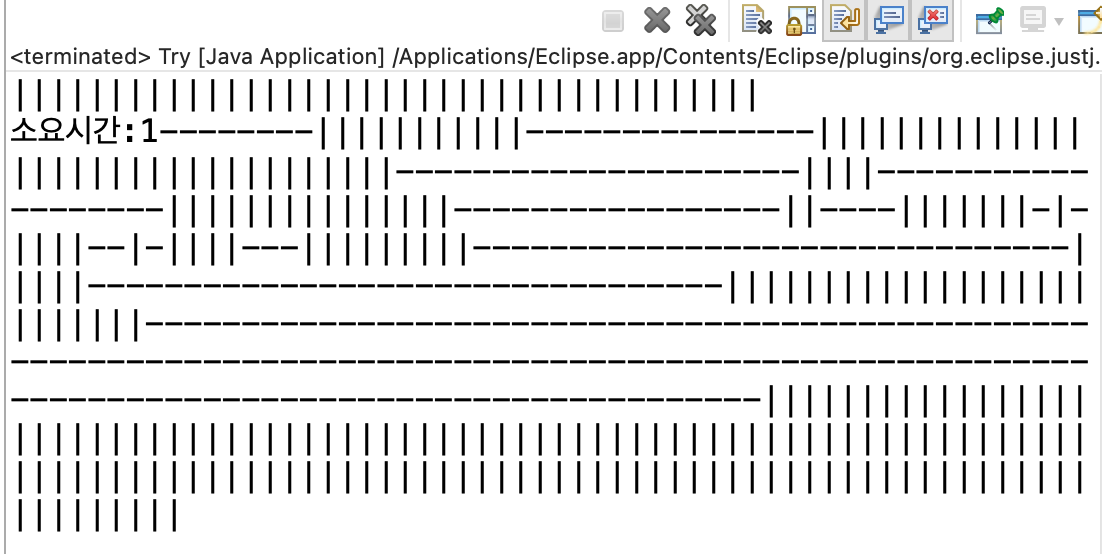
import javax.swing.JOptionPane;
public class Try {
static long startTime = 0;
public static void main(String[] args) {
ThreadEx11_1 th1 = new ThreadEx11_1();
ThreadEx11_2 th2 = new ThreadEx11_2();
th1.start();
th2.start();
startTime = System.currentTimeMillis();
// 주석처리 해보기 start
try {
th1.join(); // main쓰레드가 th1의 작업이 끝날 때까지 기다린다.
th2.join(); // main쓰레드가 th2의 작업이 끝날 때까지 기다린다.
} catch(InterruptedException e) {}
// 주석처리 해보기 end
System.out.print("소요시간:" + (System.currentTimeMillis() - Try.startTime));
}
}
class ThreadEx11_1 extends Thread {
public void run() {
for(int i=0; i < 300; i++) {
System.out.print(new String("-"));
}
} // run()
}
class ThreadEx11_2 extends Thread {
public void run() {
for(int i=0; i < 300; i++) {
System.out.print(new String("|"));
}
} // run()
}
결과값1 - ‘주석처리 해보기’ 주석처리하기 전

결과값2 - ‘주석처리 해보기’ 주석처리한 후
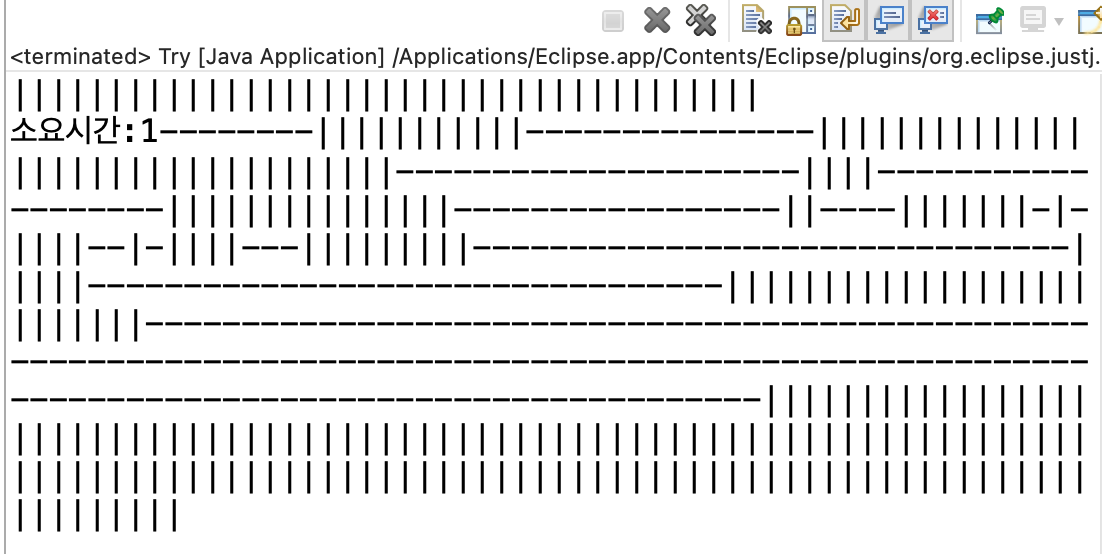
⇒ 결과값1과2를 비교해보면 소요시간이 출력되는 위치가 다른 것이 확인가능하다.
예제
더보기

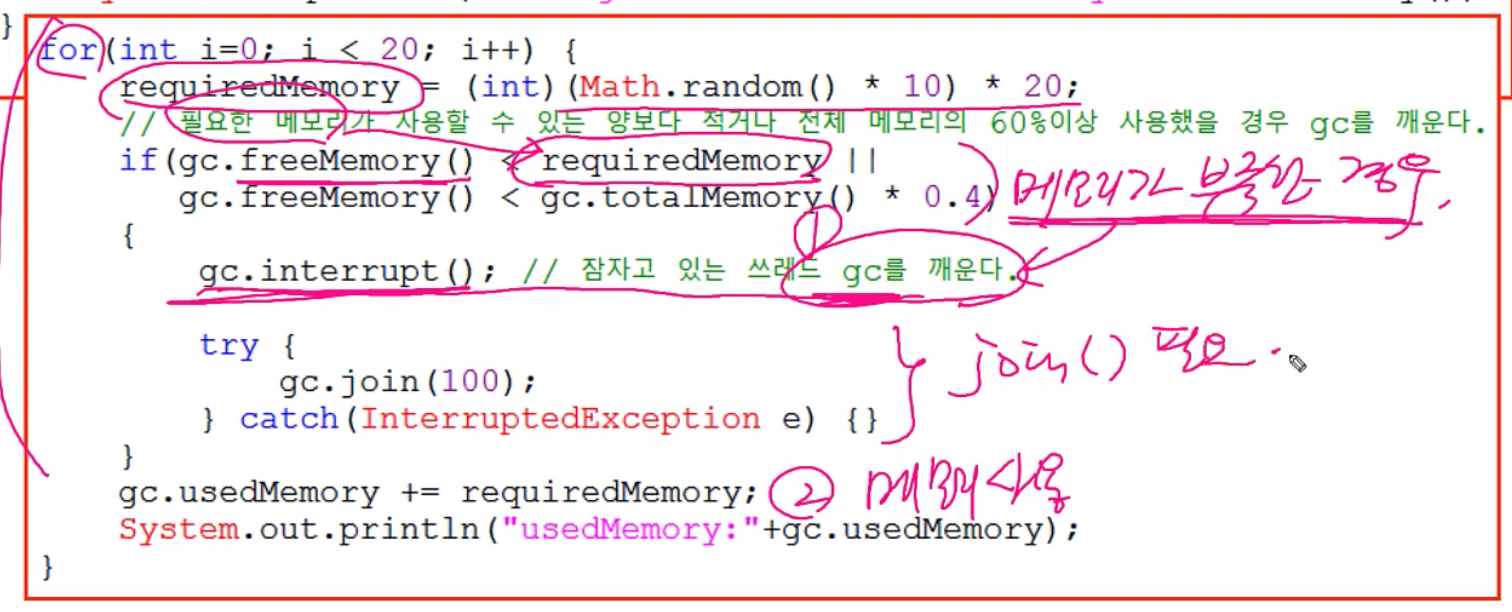

⇒ GC 가비지 컬렉터 흉내를 내보았다.
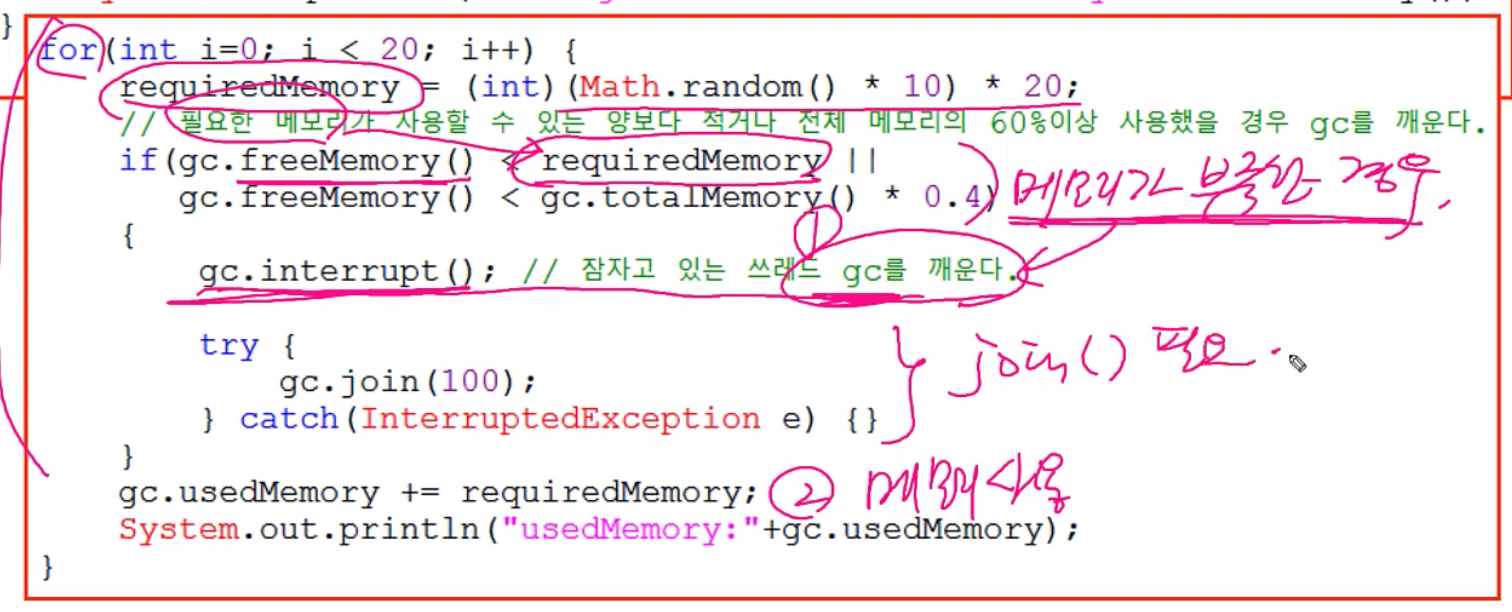
⇒ join()필요[위에코드에서 try문 추가됨.]
yield()
- 남은 시간을 다음 쓰레드에게 양보하고, 자신(현재 쓰레드)은 실행대기한다.
- yield()와 interrupt()를 적절히 사용하면, 응답성과 효율을 높일 수 있다.
'👩🏻💻 About 프로그래밍 > Java' 카테고리의 다른 글
Java wait()과 notify() (0) | 2023.12.09 |
---|---|
Java 쓰레드의 동기화(synchronization) (1) | 2023.12.09 |
Java 데몬쓰레드(deomon thread), 쓰레드의 상태 (0) | 2023.12.09 |
Java 쓰레드의 우선순위, 쓰레드 그룹 (0) | 2023.12.09 |
Java 싱글쓰레드와 멀티 쓰레드, 쓰레드의 I/O 블락킹 (1) | 2023.12.09 |
블로그의 정보
Hello 춘기's world
볼빵빵오춘기